5 Common Kubernetes Mistakes and How to Avoid Them
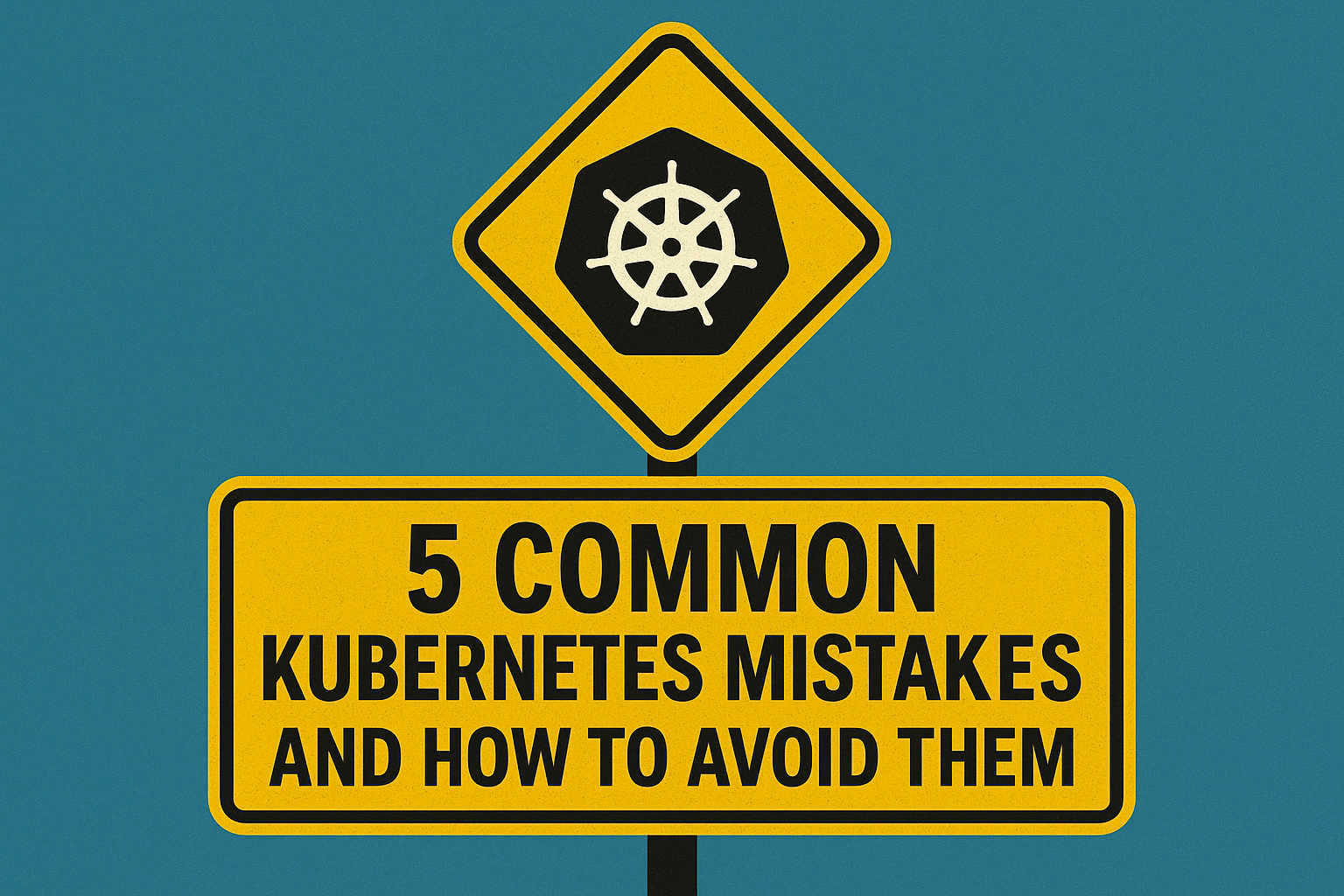
Kubernetes has revolutionized container orchestration, but with its powerful capabilities comes a steep learning curve that can leave even experienced DevOps engineers scratching their heads. As the saying goes, "With great power comes great responsibility" – and in Kubernetes' case, great opportunity for misconfiguration. Having spent countless hours troubleshooting Kubernetes clusters in production environments, I've compiled the five most common mistakes that teams make and how you can save yourself from the same headaches.
The OOMKilled Monster: Resource Limits Gone Wrong
Picture this: It's 3 AM, your phone buzzes with alerts, and you discover your production pods are repeatedly crashing with status OOMKilled
. Your morning coffee hasn't kicked in yet, but you're already diving into logs while your team frantically tries to restore services.
The culprit? Improperly configured resource limits.
Resource limits in Kubernetes define the maximum amount of compute resources a container can use, including memory and CPU. Without proper limits, applications may consume all available resources, impacting other services or causing their own termination.
When you see something like this in your pod description:
State: Running
Started: Sun, 16 Feb 2020 10:20:09 +0000
Last State: Terminated
Reason: OOMKilled
Exit Code: 137
Started: Sun, 16 Feb 2019 09:27:39 +0000
Finished: Sun, 16 Feb 2019 10:20:08 +0000
Restart Count: 7
Your pod has reached its memory limit and Kubernetes terminated it. This is Kubernetes' way of saying, "I'm going to stop you before you eat the entire buffet."
The Solution
Properly configure both resource requests and limits. While requests help with scheduling, limits prevent resource hogging at runtime:
resources:
requests:
memory: "128Mi"
cpu: "100m"
limits:
memory: "256Mi"
cpu: "500m"
For more effective resource management:
- Rightsize your applications - Monitor actual usage and adjust accordingly rather than using arbitrary values.
- Implement Quality of Service (QoS) classes - Prioritize critical workloads with appropriate QoS assignments.
- Consider Horizontal Pod Autoscaling (HPA) - Let Kubernetes automatically adjust the number of pods based on resource usage metrics.
Remember, resource limits are like speed limits – they exist for a reason, but they need to be set appropriately for your specific vehicle and road conditions.
RBAC Misconfigurations: When Permissions Go Wild
Security in Kubernetes often takes a backseat to functionality until it's too late. One of the most critical security concerns is misconfigured Role-Based Access Control (RBAC).
Consider this scenario: A well-meaning developer creates a service account for a new application with excessive permissions because "it just needs to work for now." Six months later, that application is compromised, and suddenly an attacker has access to sensitive resources across your cluster.
RBAC misconfigurations typically arise when roles and role bindings aren't properly set up, allowing users or services excessive privileges. It's like giving your intern the master key to the entire office building when they only needed access to the supply closet.
The Solution
Follow the principle of least privilege by:
- Defining specific roles with only necessary permissions:
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
name: pod-reader
namespace: default
rules:
- apiGroups: [""]
resources: ["pods"]
verbs: ["get", "list", "watch"]
- Verify permissions with the
kubectl auth can-i
command:
kubectl auth can-i --as=system:serviceaccount:: [-n ]
- Regularly audit RBAC configurations to identify and remediate potential security risks.
As the old Kubernetes adage goes: "Grant access once, verify twice, and audit regularly."
The Dreaded ImagePullBackOff: Docker Image Dilemmas
You've spent hours crafting the perfect deployment manifest, you apply it with a flourish, only to see your pods stuck in ImagePullBackOff
status. What gives?
ImagePullBackOff
occurs when Kubernetes can't pull the container image you specified. This frustrating state usually happens for one of several reasons:
- Typos in image names -
ngiinx
instead ofnginx
(we've all been there) - Using the infamous "latest" tag - Perhaps the most violated Kubernetes best practice
- Repository access issues - Authentication problems or private repository access
When troubleshooting, kubectl describe pod
will show events like:
Events:
Type Reason Age From Message
---- ------ ---- ---- -------
Normal Pulling 22s (x4 over 66s) kubelet Pulling image "ngiinx"
Warning Failed 21s (x4 over 65s) kubelet Failed to pull image "ngiinx": rpc error: code = Unknown desc = Error response from daemon: pull access denied for ngiinx, repository does not exist or may require 'docker login'
Warning Failed 21s (x4 over 65s) kubelet Error: ErrImagePull
Normal BackOff 9s (x4 over 65s) kubelet Back-off pulling image "ngiinx"
Warning Failed 9s (x4 over 65s) kubelet Error: ImagePullBackOff
The Solution
- Use specific image tags instead of
latest
- Pin to exact versions for consistency and predictability - Double-check image names - Triple-check private repository paths
- Set up proper registry credentials using Kubernetes secrets:
apiVersion: v1
kind: Secret
metadata:
name: registry-credentials
type: kubernetes.io/dockerconfigjson
data:
.dockerconfigjson:
Then reference in your pod spec:
spec:
imagePullSecrets:
- name: registry-credentials
Remember, using the latest
tag in production is like playing Russian roulette with your deployments – it might work fine until it suddenly doesn't.
CrashLoopBackOff: The Endless Restart Cycle
Few things are more frustrating than pods caught in the infamous CrashLoopBackOff state – Kubernetes' way of saying "I'll keep trying, but it's not looking good."
This error occurs when your pod starts, crashes, starts again, and crashes again in an endless cycle of failure. When you run kubectl get pods
and see:
NAME READY STATUS RESTARTS AGE
myDeployment1-89234... 1/1 Running 1 17m
myDeployment1-46964... 0/1 CrashLoopBackOff 2 1m
You know you're in for some troubleshooting fun.
Common causes include:
- Missing CMD in your Dockerfile causing immediate exit
- Port conflicts within pods
- Application errors or misconfiguration
- Resource constraints causing application crashes
The Solution
Effective troubleshooting is key:
- Check the logs with
kubectl logs
to understand why the application is crashing - Use a sleep command in your deployment temporarily to keep the container running while you investigate:
command: ["/bin/sh", "-c", "sleep 3600"]
- Check for port conflicts between containers in the same pod
- Verify environment variables and configuration settings
- Add proper liveness and readiness probes to help Kubernetes better understand your application's health:
livenessProbe:
httpGet:
path: /healthz
port: 8080
initialDelaySeconds: 15
periodSeconds: 10
readinessProbe:
httpGet:
path: /ready
port: 8080
initialDelaySeconds: 5
periodSeconds: 5
Debugging CrashLoopBackOff is like being a detective in a mystery where the main suspect keeps disappearing – persistent observation and methodical investigation are your best tools.
Network Policies: The Security Layer Everyone Forgets
In the rush to get applications deployed, network security often becomes an afterthought. Many teams simply don't implement Network Policies, leaving their clusters vulnerable to lateral movement attacks.
Without proper Network Policies, pods can communicate with any other pod in the cluster by default. It's like having a building where every door is unlocked and every room is accessible to everyone – convenient until someone with malicious intent walks in.
This oversight can be particularly dangerous in production environments where different teams' applications run side by side, or when handling sensitive data.
The Solution
Implement Network Policies to define and enforce communication rules between pods:
- Start with a default deny policy and then explicitly allow necessary traffic:
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: default-deny
spec:
podSelector: {}
policyTypes:
- Ingress
- Egress
- Create specific policies for applications that need to communicate:
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: allow-frontend-to-backend
spec:
podSelector:
matchLabels:
app: backend
ingress:
- from:
- podSelector:
matchLabels:
app: frontend
ports:
- protocol: TCP
port: 8080
- Regularly test and validate your network policies using tools like network policy validators or penetration testing
Network Policies are like the immune system of your Kubernetes cluster – you don't notice them when they're working properly, but you'll certainly notice their absence when something goes wrong.
Moving Forward With Confidence
Kubernetes can seem like a labyrinth of potential pitfalls, but understanding these common mistakes and implementing the suggested solutions will significantly improve your cluster's reliability, security, and performance.
Remember that even experienced Kubernetes practitioners make these mistakes – the difference is they learn to recognize the symptoms quickly and apply the appropriate remedies efficiently. Tools like monitoring, logging, and automated policy enforcement can help catch these issues before they impact your production environment.
As your Kubernetes journey continues, treat each error as a learning opportunity. Document the issues you encounter, share knowledge with your team, and gradually build up organizational wisdom that turns troubleshooting from an emergency response into a structured process.
After all, the mark of a true Kubernetes expert isn't never making mistakes – it's knowing exactly what to do when they inevitably occur.